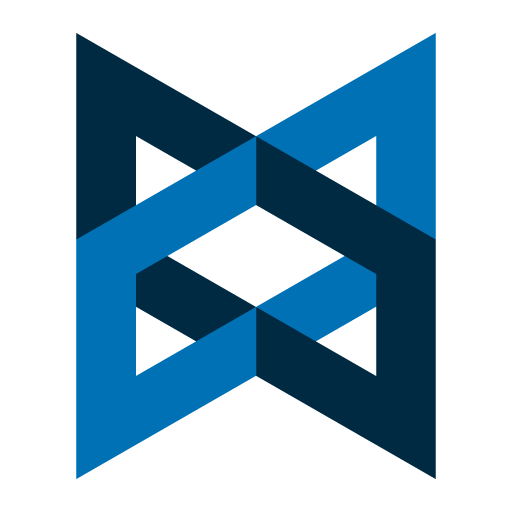
Events is a module that can be mixed in to any object, giving the object the ability to bind and trigger custom named events
Methods:
this.el properties:
window.App = { ... }; App.Photo = Backbone.Model.extend({ ... };
Models: App.Photo Collections: App.Photos Views: App.PhotoView Main router: App.Router Custom routers: App.SpecialRouter Router instance: App.router View instances: App.photoView Singleton model instances: App.photo Collection instances: App.photos
Models: App.Models.Photo Collections: App.Collections.Photos Views: App.Views.Photo Routes: App.Routes.Photo
<body> ... <script> App.photos = new Photos([ { id: 2, name: "My dog", filename: "IMG_0392.jpg" }, { id: 3, name: "Our house", filename: "IMG_0393.jpg" }, { id: 4, name: "My favorite food", filename: "IMG_0394.jpg" }, { id: 5, name: "His bag", filename: "IMG_0394.jpg" }, ... ]); </script> </body>
<script type="text/html" id="template-contact"> <div class='contact'> <strong><%= name %></strong> <span><%= email %></span> </div> </script>
window.JST = {}; window.JST['person/contact'] = _.template( "<div class='contact'><%= name %> ..." ); window.JST['person/edit'] = _.template( "<form method='post'><input type..." );
mergeMixin: (view, mixin) -> _.defaults view::, mixin _.defaults view::events, mixin.events if mixin.initialize isnt `undefined` oldInitialize = view::initialize view::initialize = -> mixin.initialize.apply this oldInitialize.apply this mergeMixin(Test.Views.PollsShow, Test.Mixin.PollsTabs)
Test.Mixin.PollsTabs = events: 'click .poll_tab_link' : 'pollTabOpen' 'click .voters_tab_link' : 'votersTabOpen' pollTabOpen: (e) -> e.preventDefault() Backbone.history.navigate("admin/polls/#{@model.get('id')}", true) votersTabOpen: (e) -> e.preventDefault() Backbone.history.navigate("admin/polls/#{@model.get('id')}/voters", true)
App.PhotoView = Backbone.View.extend({ ... }); // AVOID this! $("a.photo").click(function() { ... });
Backbone.View::destroyView = -> @remove() @unbind() @onDestroyView() if @onDestroyView
class PiroPopup.Views.PopupIndex extends Backbone.View initialize: (options) -> @collection.on 'add', @render @collection.on 'reset', @render PiroPopup.globalEvents.on "update:pivotal:data", @updatePivotalState onDestroyView: => @collection.off 'add', @render @collection.off 'reset', @render PiroPopup.globalEvents.off "update:pivotal:data", @updatePivotalState
Same as localStorage but...
<html manifest="example.appcache">... </html>
CACHE MANIFEST # Explicitly cached entries CACHE: index.html stylesheet.css images/logo.png scripts/main.js # static.html will be served if the user is offline FALLBACK: / /static.html # Resources that require the user to be online. NETWORK: *
Contact information