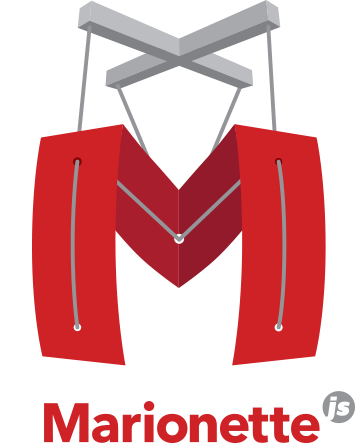
Events is a module that can be mixed in to any object, giving the object the ability to bind and trigger custom named events
Methods:
this.el properties:
window.App = { ... }; App.Photo = Backbone.Model.extend({ ... };
Models: App.Photo Collections: App.Photos Views: App.PhotoView Main router: App.Router Custom routers: App.SpecialRouter Router instance: App.router View instances: App.photoView Singleton model instances: App.photo Collection instances: App.photos
Models: App.Models.Photo Collections: App.Collections.Photos Views: App.Views.Photo Routes: App.Routes.Photo
<body> ... <script> App.photos = new Photos([ { id: 2, name: "My dog", filename: "IMG_0392.jpg" }, { id: 3, name: "Our house", filename: "IMG_0393.jpg" }, { id: 4, name: "My favorite food", filename: "IMG_0394.jpg" }, { id: 5, name: "His bag", filename: "IMG_0394.jpg" }, ... ]); </script> </body>
<script type="text/html" id="template-contact"> <div class='contact'> <strong><%= name %></strong> <span><%= email %></span> </div> </script>
window.JST = {}; window.JST['person/contact'] = _.template( "<div class='contact'><%= name %> ..." ); window.JST['person/edit'] = _.template( "<form method='post'><input type..." );
mergeMixin: (view, mixin) -> _.defaults view::, mixin _.defaults view::events, mixin.events if mixin.initialize isnt `undefined` oldInitialize = view::initialize view::initialize = -> mixin.initialize.apply this oldInitialize.apply this mergeMixin(Test.Views.PollsShow, Test.Mixin.PollsTabs)
Test.Mixin.PollsTabs = events: 'click .poll_tab_link' : 'pollTabOpen' 'click .voters_tab_link' : 'votersTabOpen' pollTabOpen: (e) -> e.preventDefault() Backbone.history.navigate("admin/polls/#{@model.get('id')}", true) votersTabOpen: (e) -> e.preventDefault() Backbone.history.navigate("admin/polls/#{@model.get('id')}/voters", true)
App.PhotoView = Backbone.View.extend({ ... }); // AVOID this! $("a.photo").click(function() { ... });
Backbone.View::destroyView = -> @remove() @unbind() @onDestroyView() if @onDestroyView
class PiroPopup.Views.PopupIndex extends Backbone.View initialize: (options) -> @collection.on 'add', @render @collection.on 'reset', @render PiroPopup.globalEvents.on "update:pivotal:data", @updatePivotalState onDestroyView: => @collection.off 'add', @render @collection.off 'reset', @render PiroPopup.globalEvents.off "update:pivotal:data", @updatePivotalState
Backbone.Marionette is a composite application library for Backbone.js that aims to simplify the construction of large scale JavaScript applications.
It is a collection of common design and implementation patterns found in the applications that we have been building with Backbone, and includes pieces inspired by composite application architectures, event-driven architectures, messaging architectures, and more.
Requirements:
(exports ? this).FalconApp = Regions: {} Routers: {} Mixins: {} Views: {} Models: {} Collections: {} Layouts: {} Utils: {} class FalconApp.Application extends Backbone.Marionette.Application onStart: (options) => # start app FalconApp.application = new FalconApp.Application $ -> FalconApp.application.start()
# templates Backbone.Marionette.Renderer.render = (template, data) -> throw "Template '#{template}' not found!" unless HandlebarsTemplates[template] HandlebarsTemplates[template](data) # custom myTemplate = _.template("<div>foo</div>") Backbone.Marionette.ItemView.extend template: myTemplate
class FalconApp.Views.InboxesItem extends Backbone.Marionette.ItemView template: 'inboxes/item' tagName: "tr" className: "state-switcher" modelEvents: 'change:name': '_setName' events: 'click .inbox_link': 'openInbox' serializeData: => _.extend @model.toJSON(), 'last_message_sent_at': @model.lastMessageSentAt() openInbox: (e) => e.preventDefault() Backbone.history.navigate("inboxes/#{@model.get('id')}/messages", { trigger: true }) _setName: => @$('span.inbox_name').text(@model.get('name')) @$('input.edit_inbox_form_name').val(@model.get('name'))
class FalconApp.Views.CompaniesList extends Backbone.Marionette.CollectionView itemView: FalconApp.Views.CompaniesItem emptyView: FalconApp.Views.CompaniesEmptyItem initialize: -> # empty onDomRefresh: => # empty
class FalconApp.Views.CompaniesItem extends Backbone.Marionette.CompositeView itemView: FalconApp.Views.InboxesItem getItemView: (item) => FalconApp.Views.InboxesItem itemViewContainer: "tbody.inboxes_list" template: 'companies/item' modelEvents: 'change:name': 'changedName' events: 'submit form.create_inbox_form': 'createInbox' initialize: -> @collection = @model.inboxes changedName: => @$('.company_name').text(@model.get('name'))
Regions provide consistent methods to manage, show and close views in your applications and layouts. They use a jQuery selector to show your views in the correct place.
MyApp.addRegions mainRegion: "#main-content" navigationRegion: "#navigation" # basic myView = new MyView() # render and display the view MyApp.mainRegion.show myView # closes the current view MyApp.mainRegion.close()
Region managers provide a consistent way to manage a number of Marionette.Region objects within an application. The RegionManager is intended to be used by other objects, to facilitate the addition, storage, retrieval, and removal of regions from that object.
rm = new Marionette.RegionManager() region = rm.addRegion("foo", "#bar") regions = rm.addRegions( baz: "#baz" quux: "ul.quux" ) regions.baz.show myView rm.removeRegion "foo"
A Layout is a hybrid of an ItemView and a collection of Region objects. They are ideal for rendering application layouts with multiple sub-regions managed by specified region managers.
class FalconApp.Layouts.Messages extends Marionette.Layout template: 'layout/messages' className: 'page_body page-body row' regions: listRegion: '.list_region' detailsRegion: '.details_region' initialize: -> # empty onDomRefresh: -> # empty
class FalconApp.Routers.Base extends Backbone.Router initialize: -> @on 'route', @_trackPageview _trackPageview: (trigger, args) => return if window._gaq is `undefined` url = Backbone.history.getFragment() url = "/#{url}" unless /^\//.test(url) window._gaq.push ['_setSiteSpeedSampleRate', 100] window._gaq.push ['_trackPageview', url] class FalconApp.Routers.Inboxes extends FalconApp.Routers.Base routes: 'inboxes': 'index' initialize: -> super() index: => companies = new FalconApp.Collections.Companies @companiesList = new FalconApp.Views.CompaniesList(collection: companies) @layout.mainRegion.show @companiesList companies.fetch()
Helps run repetitive tasks
Alternative to Rake/Cake/Make/Ant
A package manager for the web
$ npm install -g bower $ bower search $ bower search angular $ bower install $ bower install angular --save-dev $ bower list
It scaffolds out boilerplate
$ npm install -g yo $ yo jquery-boilerplate $ yo bootstrap $ npm install generator-angular -g $ yo angular
Same as localStorage but...
<html manifest="example.appcache">... </html>
CACHE MANIFEST # Explicitly cached entries CACHE: index.html stylesheet.css images/logo.png scripts/main.js # static.html will be served if the user is offline FALLBACK: / /static.html # Resources that require the user to be online. NETWORK: *
Contact information